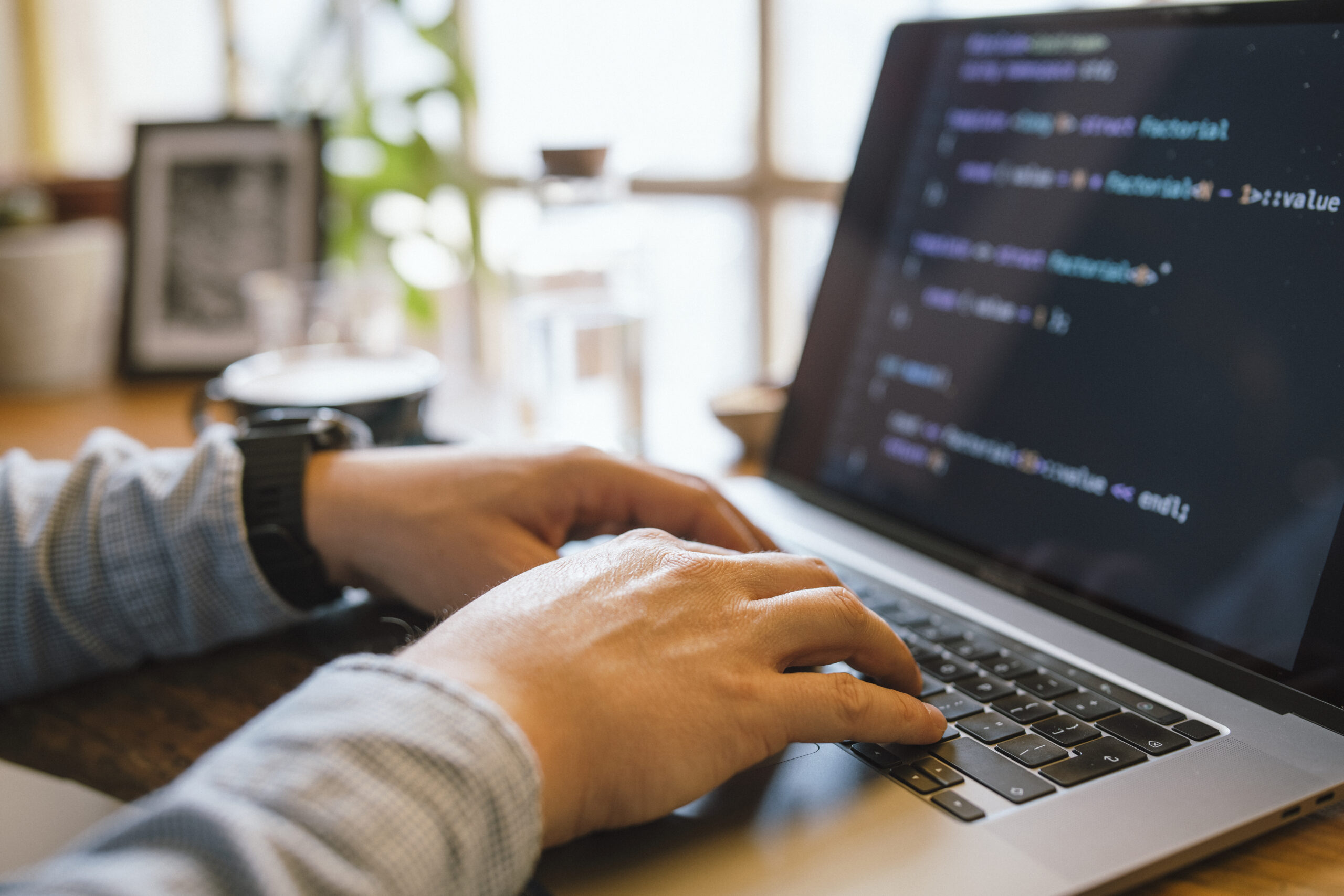
Debugging is Just about the most critical — however usually neglected — competencies in a developer’s toolkit. It isn't nearly fixing broken code; it’s about knowing how and why factors go Erroneous, and Discovering to Imagine methodically to unravel complications competently. Whether you're a beginner or a seasoned developer, sharpening your debugging skills can save several hours of irritation and radically help your efficiency. Here's many strategies to aid builders stage up their debugging game by me, Gustavo Woltmann.
Learn Your Applications
One of the fastest means builders can elevate their debugging capabilities is by mastering the applications they use everyday. When producing code is one particular Component of growth, being aware of the way to interact with it effectively all through execution is Similarly crucial. Contemporary development environments occur Outfitted with potent debugging abilities — but quite a few developers only scratch the surface of what these instruments can do.
Take, such as, an Integrated Development Ecosystem (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These applications enable you to set breakpoints, inspect the worth of variables at runtime, step as a result of code line by line, and in some cases modify code within the fly. When used accurately, they let you notice precisely how your code behaves all through execution, which happens to be a must have for tracking down elusive bugs.
Browser developer applications, including Chrome DevTools, are indispensable for entrance-finish builders. They allow you to inspect the DOM, keep an eye on community requests, check out serious-time functionality metrics, and debug JavaScript during the browser. Mastering the console, sources, and network tabs can transform irritating UI challenges into manageable duties.
For backend or process-level developers, instruments like GDB (GNU Debugger), Valgrind, or LLDB offer deep Handle around operating processes and memory administration. Learning these resources could possibly have a steeper learning curve but pays off when debugging functionality difficulties, memory leaks, or segmentation faults.
Further than your IDE or debugger, turn out to be relaxed with Model Command methods like Git to comprehend code heritage, find the exact moment bugs had been launched, and isolate problematic alterations.
In the long run, mastering your applications means going beyond default settings and shortcuts — it’s about building an intimate understanding of your advancement setting making sure that when difficulties crop up, you’re not missing at the hours of darkness. The greater you are aware of your applications, the greater time you may expend resolving the particular dilemma as an alternative to fumbling by the procedure.
Reproduce the condition
One of the most critical — and often overlooked — ways in helpful debugging is reproducing the situation. In advance of jumping in to the code or making guesses, developers require to create a dependable natural environment or circumstance in which the bug reliably seems. Devoid of reproducibility, repairing a bug gets to be a game of prospect, typically leading to squandered time and fragile code improvements.
Step one in reproducing a problem is accumulating as much context as feasible. Check with queries like: What steps brought about the issue? Which ecosystem was it in — growth, staging, or production? Are there any logs, screenshots, or mistake messages? The more element you've got, the easier it will become to isolate the exact ailments beneath which the bug takes place.
As soon as you’ve gathered ample information, endeavor to recreate the issue in your neighborhood atmosphere. This may imply inputting the exact same information, simulating very similar user interactions, or mimicking technique states. If the issue appears intermittently, look at creating automated checks that replicate the edge situations or point out transitions concerned. These assessments don't just aid expose the situation but also avert regressions Down the road.
Occasionally, The problem may very well be atmosphere-distinct — it'd happen only on specific running units, browsers, or underneath individual configurations. Utilizing equipment like Digital equipment, containerization (e.g., Docker), or cross-browser tests platforms can be instrumental in replicating this kind of bugs.
Reproducing the challenge isn’t merely a move — it’s a mindset. It demands persistence, observation, plus a methodical tactic. But as you can consistently recreate the bug, you're currently halfway to fixing it. Having a reproducible circumstance, You need to use your debugging instruments additional correctly, exam opportunity fixes properly, and connect extra Evidently with all your workforce or buyers. It turns an summary criticism right into a concrete obstacle — Which’s in which developers thrive.
Read and Understand the Mistake Messages
Mistake messages in many cases are the most worthy clues a developer has when anything goes Mistaken. As an alternative to viewing them as irritating interruptions, developers should really master to deal with error messages as direct communications within the procedure. They often tell you precisely what happened, where it transpired, and from time to time even why it took place — if you know how to interpret them.
Get started by looking at the concept carefully As well as in total. Numerous builders, particularly when under time force, glance at the main line and promptly start out generating assumptions. But deeper from the error stack or logs may well lie the correct root cause. Don’t just duplicate and paste error messages into search engines like google and yahoo — go through and understand them initially.
Break the mistake down into parts. Could it be a syntax error, a runtime exception, or possibly a logic mistake? Does it issue to a particular file and line selection? What module or operate brought on it? These queries can guideline your investigation and level you towards the responsible code.
It’s also valuable to understand the terminology in the programming language or framework you’re applying. Error messages in languages like Python, JavaScript, or Java generally follow predictable designs, and Discovering to recognize these can substantially increase your debugging procedure.
Some glitches are imprecise or generic, and in Individuals conditions, it’s essential to examine the context where the mistake occurred. Examine linked log entries, enter values, and recent adjustments while in the codebase.
Don’t ignore compiler or linter warnings either. These frequently precede more substantial difficulties and provide hints about possible bugs.
Eventually, mistake messages are certainly not your enemies—they’re your guides. Learning to interpret them properly turns chaos into clarity, aiding you pinpoint troubles speedier, cut down debugging time, and turn into a more effective and assured developer.
Use Logging Properly
Logging is Just about the most strong instruments in a very developer’s debugging toolkit. When made use of correctly, it offers serious-time insights into how an software behaves, encouraging you have an understanding of what’s going on under the hood without needing to pause execution or step through the code line by line.
A good logging strategy starts with knowing what to log and at what amount. Popular logging concentrations include things like DEBUG, Details, Alert, ERROR, and FATAL. Use DEBUG for detailed diagnostic information and facts all through progress, Details for standard activities (like effective start-ups), Alert for probable troubles that don’t break the application, Mistake for true issues, and FATAL in the event the technique can’t carry on.
Avoid flooding your logs with too much or irrelevant knowledge. A lot of logging can obscure important messages and decelerate your program. Concentrate on vital functions, state variations, enter/output values, and critical final decision factors in your code.
Structure your log messages clearly and continually. Contain context, such as timestamps, ask for IDs, and function names, so it’s simpler to trace problems in dispersed methods or multi-threaded environments. Structured logging (e.g., JSON logs) will make it even easier to parse and filter logs programmatically.
All through debugging, logs Allow you to keep track of how variables evolve, what situations are achieved, and what branches of logic are executed—all without having halting This system. They’re Specifically important in manufacturing environments where by stepping by means of code isn’t probable.
Furthermore, use logging frameworks and instruments (like Log4j, Winston, or Python’s logging module) that assistance log rotation, filtering, and integration with checking dashboards.
Finally, sensible logging is about harmony and clarity. With a properly-assumed-out logging method, you may reduce the time it will take to identify challenges, acquire deeper visibility into your apps, and Increase the General maintainability and dependability within your code.
Feel Just like a Detective
Debugging is not merely a technical activity—it's a sort of investigation. To effectively determine and correct bugs, builders will have to approach the process just like a detective fixing a thriller. This way of thinking allows stop working complicated concerns into manageable areas and adhere to clues logically to uncover the basis bring about.
Start by collecting evidence. Consider the indications of the problem: error messages, incorrect output, or functionality difficulties. The same as a detective surveys against the law scene, obtain as much relevant details as you'll be able to with no leaping to conclusions. Use logs, examination scenarios, and person experiences to piece alongside one another a transparent photo of what’s occurring.
Upcoming, kind hypotheses. Check with on your own: What may very well be resulting in this habits? Have any improvements just lately been manufactured for the codebase? Has this concern occurred before less than identical situation? The purpose is usually to narrow down possibilities and identify opportunity culprits.
Then, take a look at your theories systematically. Try and recreate the trouble inside a managed setting. In the event you suspect a selected purpose or element, isolate it and validate if The problem persists. Like a detective conducting interviews, talk to your code inquiries and let the effects direct you nearer to the truth.
Pay near interest to compact information. Bugs frequently disguise inside the the very least anticipated places—just like a lacking semicolon, an off-by-one particular mistake, or possibly a race situation. Be thorough and client, resisting the urge to patch the issue with no fully knowledge it. Temporary fixes may well hide the true trouble, only for it to resurface afterwards.
Lastly, hold notes on what you experimented with and acquired. Just as detectives log their investigations, documenting your debugging approach can save time for potential challenges and assist Some others understand your reasoning.
By pondering similar to a detective, builders can sharpen their analytical abilities, technique complications methodically, and turn out to be simpler at uncovering concealed challenges in complicated techniques.
Produce Checks
Writing exams is one of the best solutions to improve your debugging abilities and All round growth performance. Checks not only assist catch bugs early but in addition serve as a safety Internet that provides you self esteem when building variations to your codebase. A well-tested application is easier to debug because it enables you to pinpoint specifically in which and when a difficulty happens.
Begin with unit tests, which concentrate on person functions or modules. These small, isolated tests can quickly expose irrespective of whether a selected bit of logic is Doing work as predicted. Each time a check fails, you instantly know exactly where to look, significantly lessening some time expended debugging. Unit tests are especially practical for catching regression bugs—difficulties that reappear soon after Formerly becoming fixed.
Future, combine integration exams and end-to-close assessments into your workflow. These assist ensure that many portions of your application do the job jointly easily. They’re particularly handy for catching bugs that take place in complicated units with a number of components or expert services interacting. If one thing breaks, your exams can show you which Section of the pipeline failed and underneath what circumstances.
Crafting exams also forces you to definitely Feel critically regarding your code. To test a aspect appropriately, you need to be aware of its inputs, expected outputs, and edge scenarios. This degree of knowledge Normally sales opportunities to better code framework and much less bugs.
When debugging a problem, producing a failing test that reproduces the bug might be a robust first step. When the exam fails constantly, you could concentrate on repairing the bug and check out your check move when The difficulty is fixed. This method makes sure that a similar bug doesn’t return in the future.
In a nutshell, crafting tests turns debugging from a annoying guessing video game right into a structured and predictable procedure—aiding you capture additional bugs, faster and even more reliably.
Just take Breaks
When debugging a difficult difficulty, it’s easy to become immersed in the trouble—watching your display screen for several hours, seeking solution following Answer. But The most underrated debugging instruments is actually stepping absent. Getting breaks can help you reset your intellect, cut down irritation, and infrequently see The difficulty from the new perspective.
If you're too near the code for much too long, cognitive exhaustion sets in. You may perhaps get started overlooking noticeable faults or misreading code that you choose to wrote just several hours before. With this condition, your brain gets to be much less efficient at problem-resolving. A brief stroll, a coffee break, or simply switching to another undertaking for 10–15 minutes can refresh your focus. Lots of builders report obtaining the root of a problem when they've taken time and energy to disconnect, allowing their subconscious function in the history.
Breaks also support avoid burnout, especially all through extended debugging periods. Sitting down before a screen, mentally trapped, is not merely unproductive but additionally draining. Stepping absent lets you return with renewed Power in addition to a clearer frame of mind. You could suddenly detect a missing semicolon, a logic flaw, or simply a misplaced variable that eluded you ahead of.
In the event you’re trapped, a great general guideline is always to set a timer—debug actively for forty five–60 minutes, then take a five–10 moment break. Use that point to move all around, extend, or do anything unrelated to code. It may come to feel counterintuitive, especially underneath tight deadlines, nonetheless it actually contributes to faster and simpler debugging Ultimately.
In a nutshell, having breaks isn't an indication of weak spot—it’s a smart approach. It presents your brain Room to breathe, increases your perspective, and will help you steer clear read more of the tunnel vision that often blocks your development. Debugging is usually a mental puzzle, and rest is a component of resolving it.
Discover From Every single Bug
Each individual bug you experience is much more than simply A short lived setback—it's an opportunity to increase to be a developer. Whether or not it’s a syntax error, a logic flaw, or possibly a deep architectural difficulty, each one can teach you one thing worthwhile for those who take the time to reflect and evaluate what went Mistaken.
Start out by inquiring you a few important queries after the bug is settled: What induced it? Why did it go unnoticed? Could it are caught before with superior tactics like device tests, code assessments, or logging? The responses normally expose blind places as part of your workflow or being familiar with and assist you Establish much better coding patterns going ahead.
Documenting bugs can even be an outstanding practice. Hold a developer journal or keep a log where you Be aware down bugs you’ve encountered, how you solved them, and what you acquired. Eventually, you’ll begin to see designs—recurring concerns or typical mistakes—you could proactively stay clear of.
In staff environments, sharing Whatever you've realized from a bug with all your friends could be Particularly impressive. Irrespective of whether it’s by way of a Slack message, a brief publish-up, or a quick awareness-sharing session, supporting Other individuals steer clear of the similar concern boosts team performance and cultivates a more powerful Discovering lifestyle.
Much more importantly, viewing bugs as classes shifts your attitude from frustration to curiosity. In place of dreading bugs, you’ll commence appreciating them as critical areas of your improvement journey. In spite of everything, a number of the best developers are not the ones who generate best code, but those who continually learn from their problems.
In the end, Every single bug you deal with adds a whole new layer to your ability established. So next time you squash a bug, take a instant to reflect—you’ll appear absent a smarter, much more capable developer thanks to it.
Conclusion
Strengthening your debugging competencies requires time, exercise, and tolerance — however the payoff is big. It will make you a more effective, self-confident, and able developer. The next time you are knee-deep in the mysterious bug, try to remember: debugging isn’t a chore — it’s an opportunity to become greater at That which you do.